Extract simple product sku when adding product to wishlist in Magento 2
Our team was trying to send simple / child product to Facebook as part of the event tracking but it took us a while to figure out how to extract the information like simple product name, sku and price. Because by default it gives you only product ID of the configurable / parent product along with variant information like size and colour IDs but no information related to the simple / child product.
There are following wishlist events available which can be used to retrieve wishlist item(s) which can further be used to retrieve product ID of the simple / child product -:
- wishlist_add_product $this->_eventManager->dispatch(‘wishlist_add_product’,[‘wishlist’ => $wishlist, ‘product’ => $product, ‘item’ => $result]);
- wishlist_product_add_after $this->_eventManager->dispatch(‘wishlist_product_add_after’, [‘items’ => $items]);
- wishlist_add_item$this->_eventManager->dispatch(‘wishlist_add_item’, [‘item’ => $item]);
wishlist_add_product and wishlist_add_item will give you item level information which will help you extract simple product information while wishlist_product_add_after will give you information about all the items which have been added to the wishlist
In this post, we will use wishlist_add_product to retrieve simple product SKU which was added to the wishlist by the customer
Step 1: Create events.xml under ScommerceCustometcfrontend folder with the following code -:
<br> <?xml version="1.0" encoding="UTF-8"?><br> <config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Event/etc/events.xsd"><br> <event name="wishlist_add_product"><br> <observer name="scommerce_custom_wishlist_add_product" instance="ScommerceCustomModelObserverWishlistAddProduct" /><br> </event><br> </config><br>
Step 2: Create WishlistAddProduct.php under ScommerceCustomModelObserver folder with the following code -:
<br> <?php<br> /**<br> * Copyright © 2015 Scommerce Mage. All rights reserved.<br> * See COPYING.txt for license details.<br> */<br> namespace ScommerceCustomModelObserver;</p> <p>use MagentoFrameworkEventObserver as EventObserver;<br> use MagentoFrameworkEventObserverInterface;</p> <p>class WishlistAddProduct implements ObserverInterface<br> {</p> <p>/**<br> * @var MagentoFrameworkObjectManagerInterface<br> */<br> protected $_objectManager;</p> <p>/**<br> * @param MagentoFrameworkObjectManagerInterface $objectmanager<br> */<br> public function __construct(MagentoFrameworkObjectManagerInterface $objectmanager)<br> {<br> $this->_objectManager = $objectmanager;<br> }</p> <p>public function execute(EventObserver $observer)<br> {<br> $productId = $observer->getItem()->getOptionByCode('simple_product')->getValue();<br> $product = $this->_objectManager->create('MagentoCatalogModelProduct')<br> ->load($productId);</p> <p>if (!$product->getId()) {<br> return;<br> }<br> return $product->getSku();<br> }<br> }
Below is the piece of code which will help you retrieve product id of the simple product which was choosen by the customer while adding product to wishlist.
$observer->getItem()->getOptionByCode(‘simple_product’)->getValue();
That’s it, Hope this article helped you in some way. Please leave us your comment and let us know what do you think? Thanks.
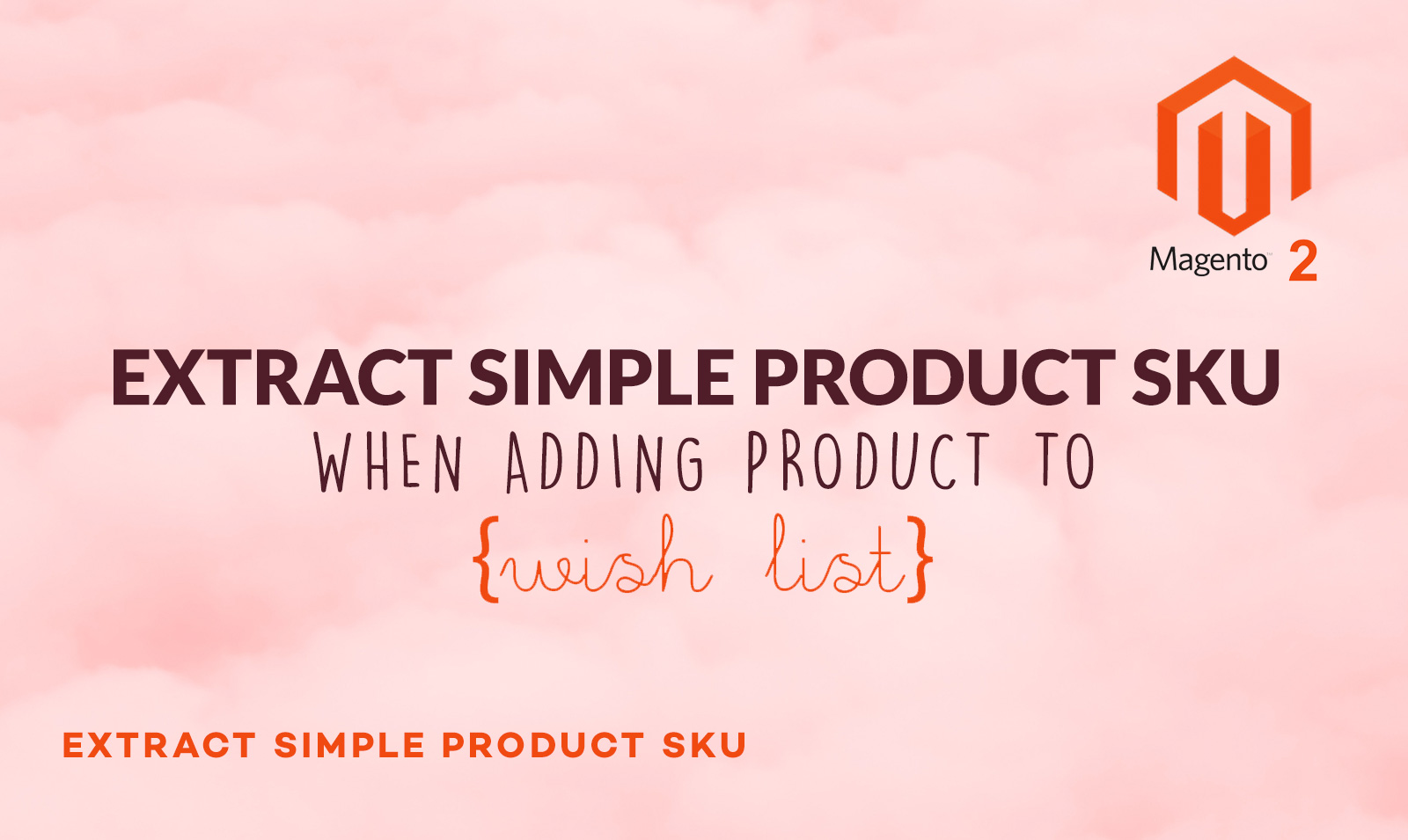